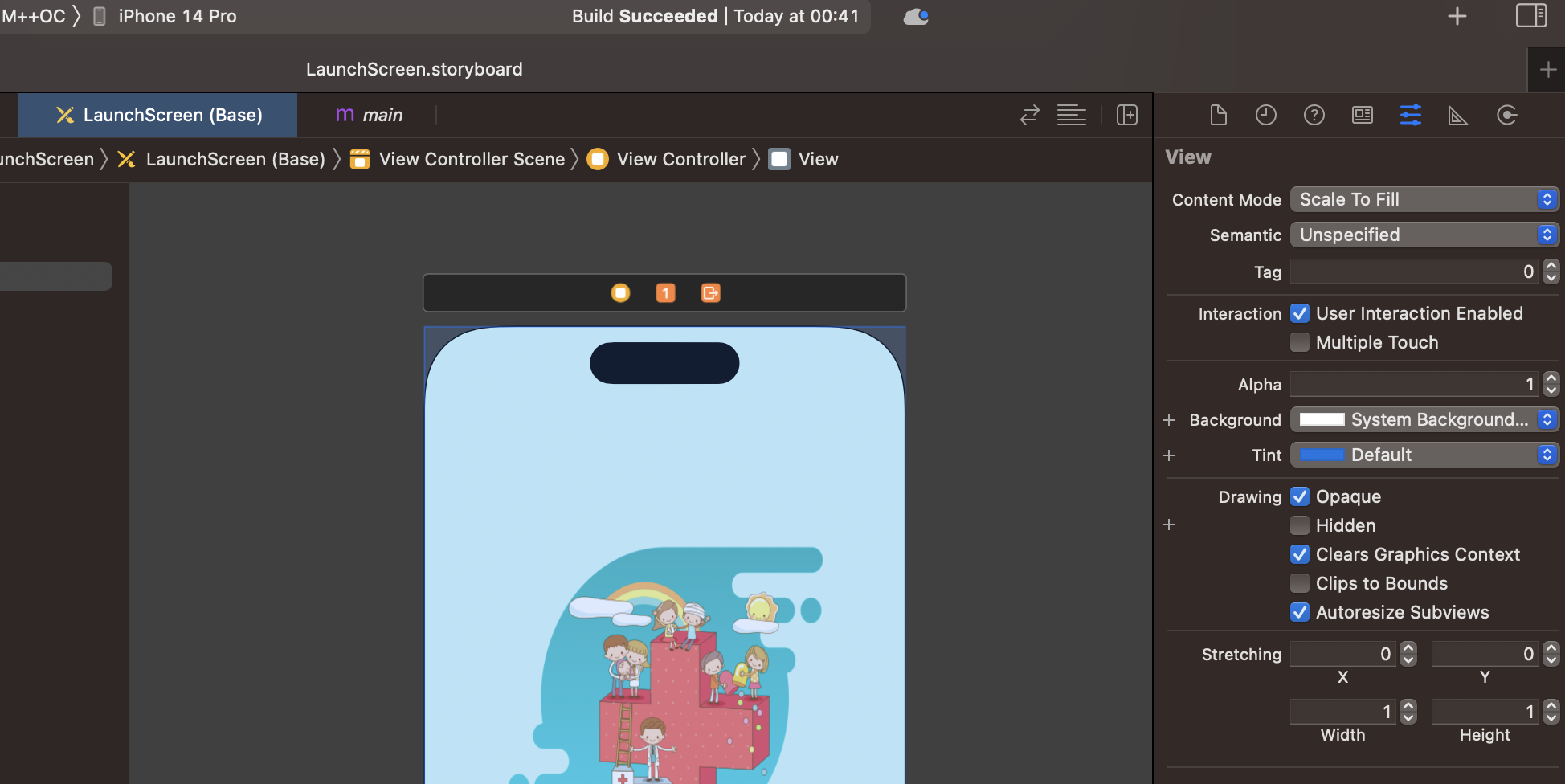
UIImageView 是IOS图片控件。点击右上角 加号。可以打开控件窗口
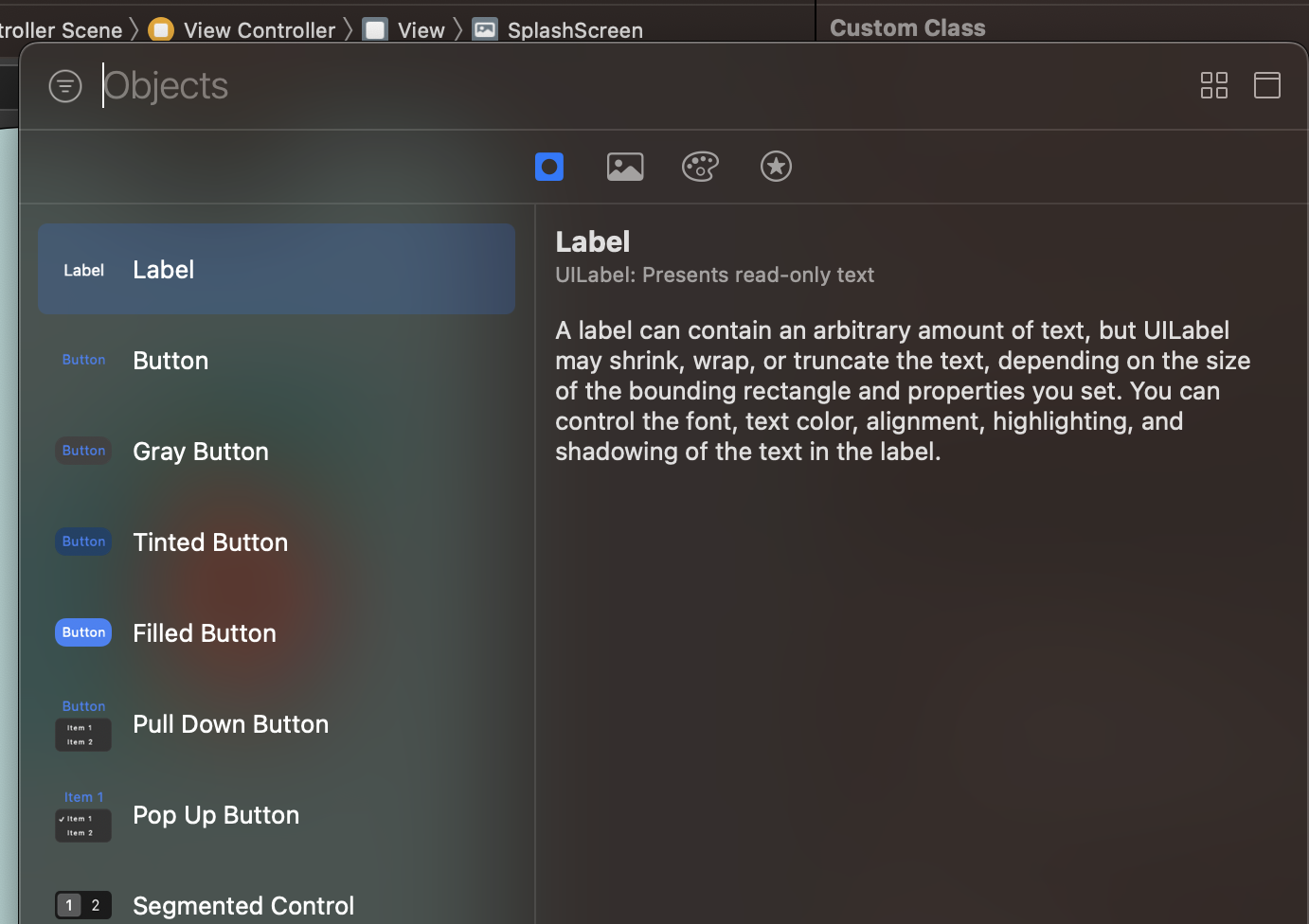
绑定图片到UIImageView
点击UIImageView。右上角image选择图片绑定
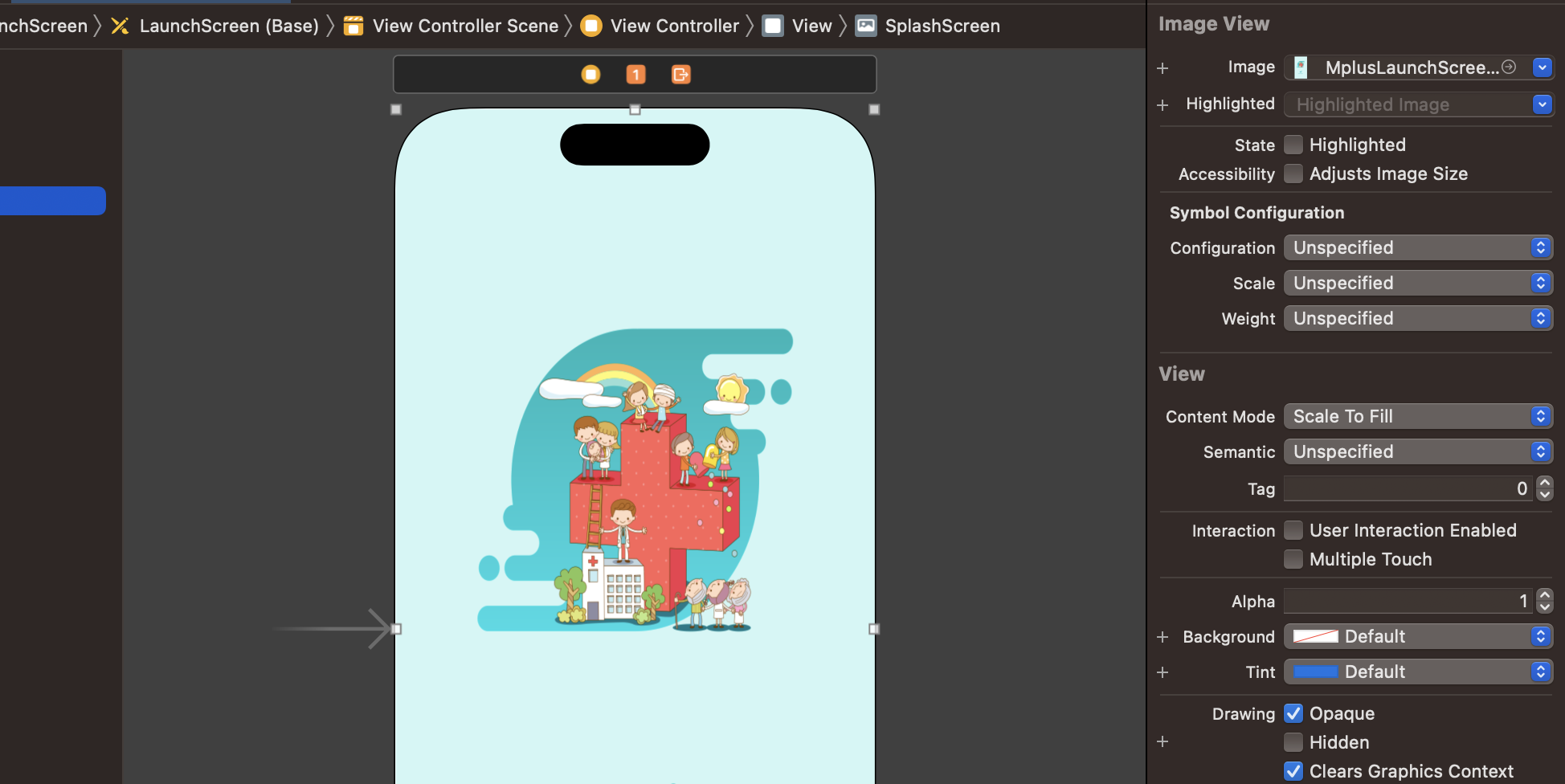
UIImageView 是IOS图片控件。点击右上角 加号。可以打开控件窗口
绑定图片到UIImageView
点击UIImageView。右上角image选择图片绑定
Saler.h //头文件声明声明对外公开的方法 声明公共属性和私有属性
@interface Saler : NSObject {
@private NSThread *thread;
}
@property NSNumber *ticket;
-(void) startSale;
@end
Saler.m //声明具体方法实现 init为默认的构造函数
#import "Saler.h"
@implementation Saler
-(Saler*) init{
self = [super init];
NSLog(@"init saler");
self.ticket = [NSNumber numberWithInt:20];
//公共属性用.访问
return self;
}
-(void) startSale{
NSThread *thread = [NSThread new];
self->thread = thread;
//私有属性用->访问
[thread initWithTarget:self selector:@selector(run) object:nil];
[thread start];
}
-(void) run{
NSLog(@"running");
}
@end
NSNetService //socket server
NSPort
子类有NSSocketPort(ip地址 + 端口) //socket client
子类有NSMachPort(应用消息通讯) 多线程通过此端口应用通讯
子类有NSMessagePort (NSMachPort对消息的封装)
但是 objective-c 有
NSConnection
NSURL
用来处理http请求 file请求 ftp请求 都是一些比较上层的应用层协议
NSURL *url = [NSURL URLWithString:@"https://www.baidu.com"];
//2、创建请求(Request)对象(默认为GET请求);
NSURLRequest *requst = [[NSURLRequest alloc]initWithURL:url];
//3、发送请求
/*
第一个参数:请求对象
第二个参数:响应头
第三个参数:错误信息
返回值:NSData类型,响应体信息
*/
NSError *error = nil;
NSURLResponse *response = nil;
//发送同步请求(sendSynchronousRequest)
NSData *data = [NSURLConnection sendSynchronousRequest:requst returningResponse:&response error:&error];
NSLog(@"connection data:%@",[[NSString alloc]initWithData:data encoding:NSUTF8StringEncoding]);
NSLog(@"connection error:%@",error);
文件输入流
NSMutableData *data = [NSMutableData new];
NSInputStream *inputStream = [NSInputStream inputStreamWithFileAtPath:@"/Users/dengfang/111.txt"];
[inputStream open];
NSLog(@"数据读取中...");
while(1){
uint8_t buf[1024];
NSInteger readLength = [inputStream read:buf maxLength:1024];
if (readLength > 0) {
[data appendBytes:buf length:readLength];
} else {
NSLog(@"未读取到数据");
break;
}
}
NSLog(@"%@" ,[data base64EncodedStringWithOptions:nil]);
}
文件输出流
NSOutputStream *ouputStream = [NSOutputStream outputStreamToFileAtPath:@"/Users/dengfang/222.txt" append:false];
[ouputStream open];
[ouputStream write:data.bytes maxLength:data.length];
[ouputStream close];
NSThread 为objective-c 的多线程对象
#import "Saler.h"
@implementation Saler
-(Saler*) init{
self = [super init];
NSLog(@"init saler");
self.ticket = [NSNumber numberWithInt:20];
return self;
}
-(void) startSale{
NSThread *thread = [NSThread new];
self->thread = thread;
[thread initWithTarget:self selector:@selector(run) object:nil];
//该访问指明调用self.run 方法作为线程运行代码
[thread start];
}
-(void) run{
NSLog(@"running");
}
@end
main 函数
#import <Foundation/Foundation.h>
#import "Saler.h"
int main(int argc, const char * argv[]) {
@autoreleasepool {
Saler* saler1 = [Saler new];
Saler* saler2 = [Saler new];
[saler1 startSale];
[saler2 startSale];
NSLog(@"starting");
}
return 0;
}
console output
2023-04-02 20:58:33.568419+0800 CMD[2079:186178] init saler
2023-04-02 20:58:33.568662+0800 CMD[2079:186178] init saler
2023-04-02 20:58:33.569828+0800 CMD[2079:186178] starting
2023-04-02 20:58:33.570763+0800 CMD[2079:186233] running
2023-04-02 20:58:33.570776+0800 CMD[2079:186234] running
Program ended with exit code: 0
NSDate
NSDate *date = [NSDate new]; //默认为当前日期
//NSDate *date = [NSDate date]; //调用date方法也可以
NSLog(@"print date is %@",date);
dateFormat 格式化输出
NSDateFormatter *formatter = [NSDateFormatter new];
formatter.dateFormat = @"yyyy-MM-dd HH:mm:ss z";
//[formatter setDateFormat:@"yyyy-MM-dd HH:mm:ss z"];
NSString *str = [formatter stringFromDate:date];
NSLog(@"print date is %@",str);
dateFormat 反格式化
NSString *dateString = @"2023-04-02 11:33:04";
NSDateFormatter *formatter = [NSDateFormatter new];
[formatter setDateFormat:@"yyyy-MM-dd HH:mm:ss"];
NSDate *date = [formatter dateFromString:dateString];
NSLog(@"print date is %@",date);
objective-c有自己封装的集合类 字符串类 和 数字类 大多数有不可变和可变之分
NSData 二进制byte数组
NSValue C语言基本数据类型 结构体 的NS封装
NSString /NSMutableString 字符串类
NSNumber 基本数字类
NSDecimalNumber 高精度数字类
NSArray/NSMutableArray 数组
NSDictionary/NSMutableDictionary 字典
NSSet/NSMutableSet 无序集合
https://blog.csdn.net/pishum/article/details/125152886
解决苹果开发者账户 逾期问题 (xcode不允许编译)
苹果官方文档
NSLog 控制台输出
NSLog(NSString *format, …)
objective-c 文件系统依赖NSFileManager
objective-c file对象 为NSFileHandle
NSFileManager 返回的文件路径对象都是NSString
fileManager.currentDirectoryPath; //返回当前进程目录
BOOL isSuccess = [fileManager createFileAtPath:path contents:nil attributes:nil]; // 创建文件
BOOL isDirectorySuccess = [fileManager createDirectoryAtPath:directory withIntermediateDirectories:true attributes:nil error:nil]; //创建文件夹
BOOL isRemoveSuccess = [fileManager removeItemAtPath:path error:nil];//删除文件或者文件夹
以下是读写文件文件 和文件对象操作
NSString 对应字符串
NSData 对应byte数组
NSFileHandle 对应文件对象
NSString *homePath = NSHomeDirectory( );
NSString *sourcePath = [homePath stringByAppendingPathConmpone:@"testfile.text"];
NSFileHandle *fielHandle = [NSFileHandle fileHandleForUpdatingAtPath:sourcePath]; //创建文件对象
[fileHandle seekToEndOfFile]; 将节点跳到文件的末尾
NSString *str = @"追加的数据"
NSData* stringData = [str dataUsingEncoding:NSUTF8StringEncoding];
[fileHandle writeData:stringData]; //追加写入NSData数据
[fileHandle closeFile]; //关闭文件对象
通过fileManager 在创建文件时写入NSData
NSString *content = @"content2";
NSData *data = [content dataUsingEncoding:NSUTF8StringEncoding];
BOOL isSuccess = [fileManager createFileAtPath:path contents:data attributes:nil];
if (isSuccess) {
NSLog(@"file created");
}else{
NSLog(@"file not created");
}
通过NSMutableString 写入文件
NSMutableString *content = [NSMutableString new];
[content appendString:@"helloworld"];
NSString *homePath = NSHomeDirectory();
NSString *path = [homePath stringByAppendingPathComponent: @"myfile.txt"];
BOOL isWriteSuccess = [content writeToFile:path atomically:true encoding:NSUTF8StringEncoding error:nil];
if (isWriteSuccess) {
NSLog(@"content writed");
}else{
NSLog(@"content not writed");
}
通过NSData 写入文件
NSString *content = @"helloworldNSdata2";
NSString *homePath = NSHomeDirectory();
NSString *path = [homePath stringByAppendingPathComponent: @"myfile.txt"];
NSData *data = [content dataUsingEncoding:NSUTF8StringEncoding];
BOOL isWriteSuccess = [data writeToFile:path atomically:true];
if (isWriteSuccess) {
NSLog(@"content writed");
}else{
NSLog(@"content not writed");
}
以下是流操作 NSInputStream和NSOutputStream
用文件流说明流章节比较复杂 下个章节单独说明